Concept:
As I was brainstorming what kind of generative art I wanted to portray, I thought of these few images/visuals that gave a more futuristic vibe while also playing around with the lines, waves, etc.:
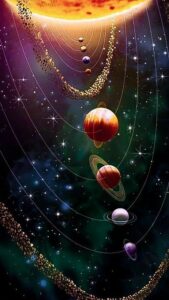
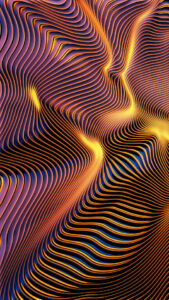
link 1, link 2, link 3, link 4, link 5
I have always been a space fan, so I decided that I wanted my concept to be futuristic, bright pop of colors, etc., but I also wanted to portray the space in a more creative way. However, I wanted to incorporate some creativity into my project rather than simply recreating the space, so I wanted to add a person/person’s body part so that it shows our connection to the universe. This led me to think of two hands controlling the galaxy/planets inside the palms of the person’s hands, so I came up with the following sketch:
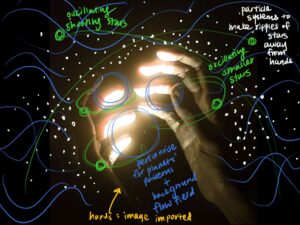
But as for the coding aspect, I decided to use the following skills we learned in class, such as:
- using perlin noise to create the surface of the planets as well as the background
- particle systems to make “ripples” of stars that are placed around the planets/hands that will scatter into the universe in a ripple pattern
- oscillation for the smaller stars/planets that will rotate around the set of hands as well as “shooting stars”
As much as it took for me to decide on a concept, once I had a vision of what I wanted to code, I was ready to move forward as quick as possible.
Design/Progress of my Code:
<Flow Field>
First, I created the flow field background so that it’ll signify the movement of the river/water, which I did so after reviewing how to create flow fields with perlin noise by watching this video.
A specific aspect that I am proud of was the “const num = ” part, because as simple as this is, I couldn’t figure out how to reduce the number of particle strokes in the background.
let particles = [];
const num = 150;
const noiseScale = 0.01 / 2;
Another part that I adjusted a lot was the following code, because for some reason the code kept glitching and wasn’t showing me a consistent transparency of the strokes when they were cleared and redrawn on canvas.
stroke(255, 60); // adjust the last number for the transparency of the strokes
strokeWeight(7); // adjust the thickness of the strokes
clear();
As for the main code that draws the flow field, I used the following code:
for (let i = 0; i < num; i++) {
let p = particles[i];
point(p.x, p.y);
let n = noise(
p.x * noiseScale,
p.y * noiseScale,
frameCount * noiseScale * noiseScale
);
let a = TAU * n;
p.x += cos(a);
p.y += sin(a);
if (!onScreen(p)) {
p.x = random(width);
p.y = random(height);
}
First, I made a loop for the particles array that runs for ‘num’ times, and I also set the position and how I want to draw the particle points so that it creates a visible trail on canvas. Then I calculated the angle a for each particle’s movement using perlin noise, as well as setting the particles so that if they go off screen, they will be reset at a random location.
<Circle with Perlin Noise>
A highlight of my circle was the following code, which basically enabled me to draw the perlin noise pattern just INSIDE the circle only by checking if the pixel is inside the circle. I also adjusted the values inside the let gray = map(); part of the code to make it into my desired shade of gray.
// check if the pixel = inside the circle
if (d < radius) {
// calculate Perlin noise value based on pixel's position
let noiseValue = noise(x * 0.01, y * 0.01);
// map the noise value to control the grayscale color
let gray = map(noiseValue, 0, 0.2, 50, 120);
stroke(gray); // stroke color
point(x, y); // draw a pixel at calculated position
}
Finally, for both the circle and the background flow field, I used the following code to make it so that every time the user presses the screen with the mouse, the direction of the particles AND the perlin noise pattern in the circle will be randomly redirected/refreshed.
function mouseReleased() {
noiseSeed(millis());
}
Here are a few different screenshots of my canvas so far:
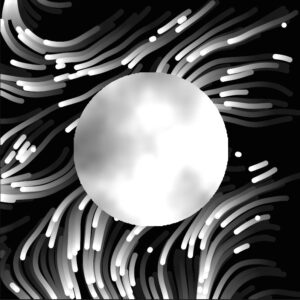
And this is what I have so far:
Uncertain Element:
For the ripples, I watched this link 1 to see what kind of ripple effect I wanted to create, and decided that small particles expanding in circles will be suitable, for I really liked the examples we did with the particles system chapter. I haven’t had the time to work on the ripples yet, though, and it’s still yet to be decided.
I’d also like to experiment with the audio and how it can affect different elements in my canvas, which is a rather scary area because I’ve never tried anything like that before.
Future Directions:
I think I will continue experimenting with different skills such as oscillation, particles system, etc. that I wanted to initially add and see if I like any accidental steps that I discover along the way. I need to create more of the perlin noise patterned planets, and also adjust their sizes and the canvas first as well as importing the hands before moving onto the other elements.