For the first week’s project, I decided to implement a self-avoidant walk and boost it with changes in HSB according to the walker’s position on the 2D plane.
THE SKETCH:
https://editor.p5js.org/llluka/sketches/iYn-Ng2tv
The canvas is split into a grid of cells, with each square indicating a possible location for the self-avoiding random walker. As a result, a 2D array was formed to track the walker’s movement, with every element corresponding to a cell on the canvas. Such structure ensures that the walker does not revisit previously occupied cells and moves in an intricate pattern on the canvas. The walker begins its movement from the center of the canvas, and its movement is decided randomly. The path is finite, depending on how fast the walker ends up in a position where all of the neighbor cells are already occupied.
The coloring of the walker’s path is dynamically generated based on the walker’s position, both x and y coordinates, creating different gradients of hue, saturation, and brightness on different parts of the canvas. Hue is influenced by both x and y, while saturation varies vertically, and brightness varies horizontally. Here is the code I used to achieve that:
// Influencing the hue based on both x and y positions
let hue = map(x + y, 0, cols + rows, 0, 360);
// Adjusting the saturation based on y so that the saturation varies vertically on the canvas.
let saturation = map(y, 0, rows, 100, 255);
// Adjusting brigthness based on x so that the brightness varies horizonatally on the canvas
let brightness = map(x, 0, cols, 100, 255);
// Set the stroke color using HSB color mode with adjusted saturation and brightness
stroke(hue, saturation, brightness, 200);
For future advancements for this project, I would imagine implementing a backtracking algorithm that would allow the walker to backtrack and retrace its steps when it encounters a dead end. In such a way the walker’s journey could be extended, resulting in longer patterns. Nevertheless, this project was a great opportunity to practice 2D arrays. I remember making board games in my Intro to Computer Science class, and it was exciting to implement the same structures but in another language.
Here are a few images of the more beautiful patterns that occurred:
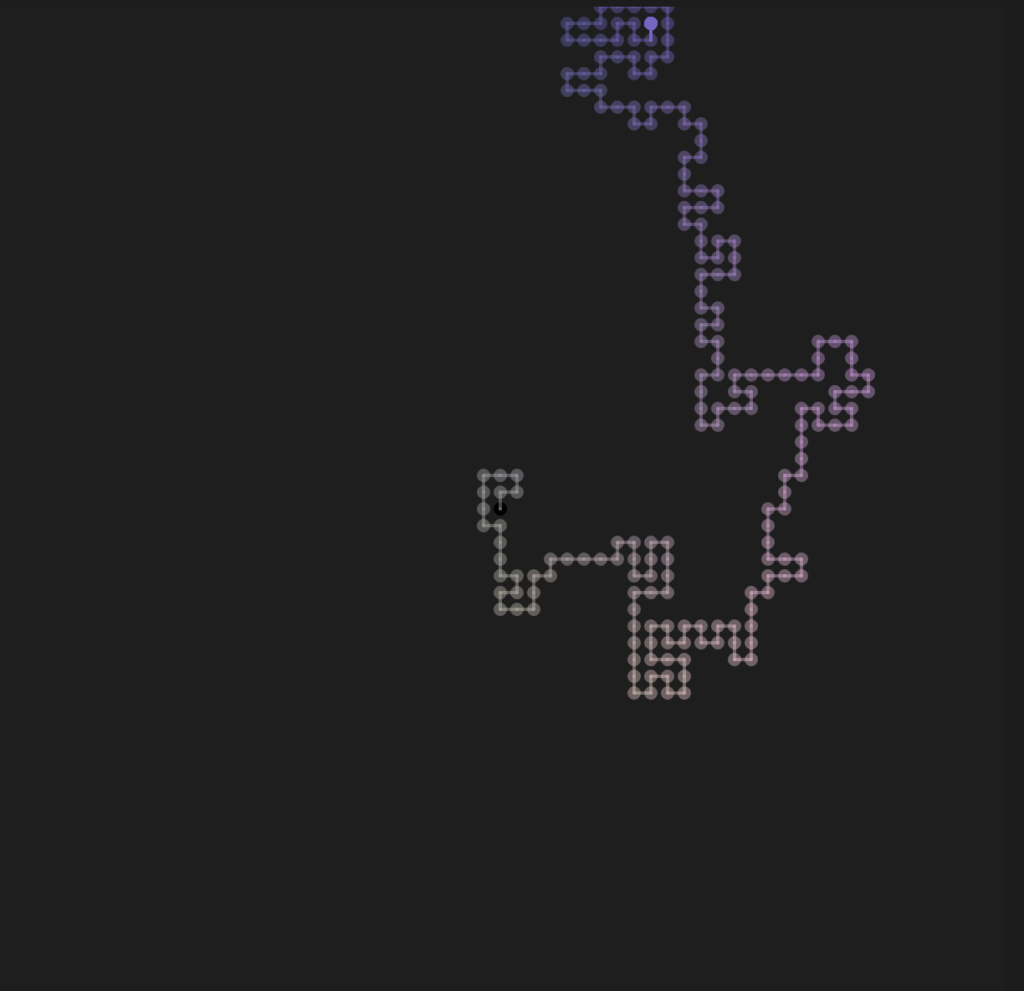
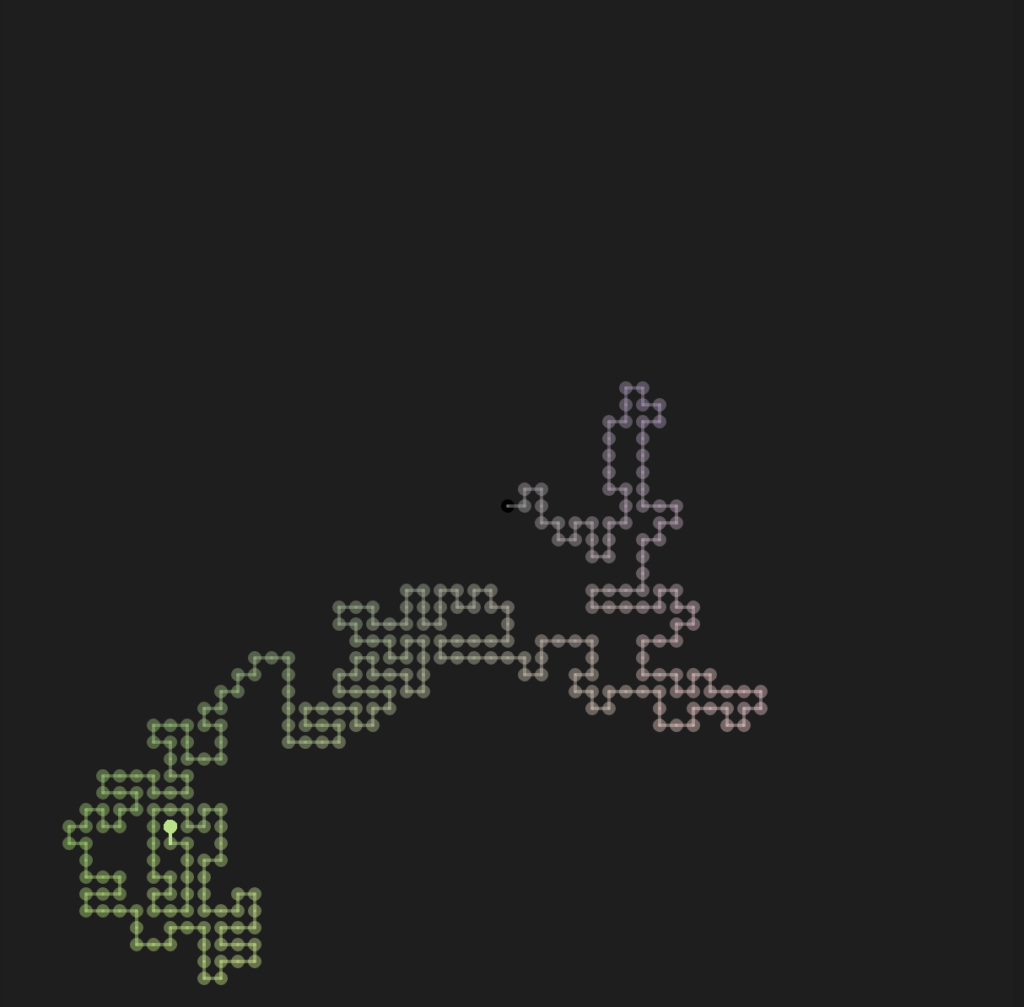